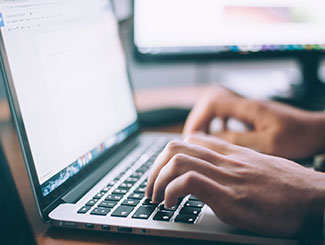
Hybrid applications – Data access layer – Web API
4 May 2015
Background
In this post I will present how to create a simple web application with an ASP.NET Web API back end.
We will use the Entity Framework ORM in data access layer to communicate with sample database. The retrieved data will be presented on simple HTML web page.
Below are the main points for the sample app:
-
Create a data model in Entity Framework 6
-
Generate a database from the EF data model
-
Create some Web API REST service
-
Display sample data in web page
Introduction
Web API
To better understand of data access layer in hybrid applications it is necessary to make some brief introduction of Web API services.
Web API is the communication interface using HTTP protocol and JSON or XML language. Generally the JSON is the light data exchange text format and it base on subset of Java Script language. It is possible to use CRUD methods of HTTP protocol:
Method |
Action |
POST |
Create |
GET |
Read |
PUT |
Update |
DELETE |
Delete |
Web API lets communication between users and system localized on the server. It is used by many of devices based on various platforms.
ASP.NET Web API
ASP.NET Web API is a framework to building web APIs on top of the .NET Framework. Generally it is helpful to creating HTTP services and it can be used by various clients from internet browsers to mobile devices. ASP.NET Web API is ideal platform to build REST applications based on .NET Framework.
ASP.NET Web API service class inherits ApiController class which provides some helpful framework methods and properties.
Let’s assume that we have some sample service class contains GetAd(int id) method and in this case:
- Framework map this method to calling GET method and pass value of id parameter in URL address
- Our task is implement GetAd method body
- Framework response to the client (object serialization to XML or JSON)
ASP.NET Web API services are usually used in:
- Rich client web applications
- SPA (Single – page applications)
- Mobile device applications
Sample Visual Studio 2013 solution
To demonstrate how to create and use the ASP.NET Web API service we will create some VS solution.
In this solution we can find:
- Entity Framework talks to the database
- ASP.NET Web API use the data model to get some data
- ASP.NET MVC creates the HTML page
Create the project
In Visual Studio select File -> New -> Project.
In the New Project window select Web (on the left pane) and then ASP.NET Web Application.
Put some project name (e.g. PortalOGService) and click OK.
In the next window select Web API template, uncheck Host in the cloud, click Change Authentication button and select No Authentication radio button. Click OK to save changes and OK again to create our solution.
Add the project with data model
Select PortalOGService solution, right click and select Add -> New Project.
In the Add New Project window select Class Library template.
Put some project name (e.g. PortalOGService.Data) and click OK.
In the new project add the new folder and name it Model.
Below is the sample screen of our solution:
Select Model folder, right click and select Add -> New Item.
In the Add New Item window select ADO.NET Entity Data Model.
Put some model name (e.g. PortalOGModel) and click OK.
In the Entity Data Model Wizard window select Empty EF Designer model and click Finish.
The next step is define desired data model of our sample application.
Below is the sample data model screen of PortalOGService solution:
At the end it is possibility to generate the database from model and create some model classes which can be used while Web API controllers creating.
Add Web API Controllers
In the previous section we created a data model which provide some model classes.
It is necessary to add reference to the PortalOGService.Data in PortalOGService project to get access to the model classes.
In the next step we can add Web API controllers to perform CRUD operations (create, read, update and delete) on those entities.
The controllers will use the Entity Framework to communicate with database layer.
In the Visual Studio, select the Controllers folder (in the main PortalOGService project), right click and select Add -> Controller…
In the Add Scaffold window select Web API Controller with actions, using Entity Framework and click Add.
Next, in the Add Controller window choose the Model class (some entity from the data model), create a new Data context class (button + clicking) and check the Use async controller actions check box.
Below the screen of Add Controller window:
Please note that the new controller is a class inherits ApiController framework class.
The controller contains a set of methods that correspond to the CRUD operations:
Method |
CRUD operations |
Description |
GetAds() |
GET api/Ads |
Get a collection of Ad |
GetAd(int id) |
GET api/Ads/{id} |
Get an Ad by identifier |
PutAd(int id, Ad ad) |
PUT api/Ads/ {id} |
Edit an existing Ad (by identifier) |
PostAd(Ad ad) |
POST api/Ads |
Create a new Ad |
DeleteAd(int id) |
DELETE api/Ads/{id} |
Delete an Ad (by identifier) |
Create Data Transfer Objects (DTO)
For this moment we have Web API service that exposes database entities to the client. The client has access directly to the entities which corresponds to the database tables.
It is good practice to create some kind of objects called data transfer object. A DTO is an object that defines how the data will be send over the network and how it will be presented to the client.
The most common reasons for using DTOs are:
- Hide some unnecessary properties from the client
- Change property names (more user friendly)
- Remove circular references (it is connected with relations between database tables)
- Separate service layer from the database layer
To create some DTO, select Models folder (in the main PortalOGService project), right click and Add -> Class.
Put some name (e.g. AdDTO) and define format of the new object.
The new DTO class can be like below:
The next step is change of initial version of AdsController class.
We can replace the GET method with version that return DTOs.
Initial version of GET method:
Final version of GET method (with DTO):
Using Web API service
Right now we have completed Web API service to handling Ads objects and communicate with database via Entity Framework.
The next step is create simple client for the application with using HTML.
The client can be created for example with using Java Script and the Knockout library.
After install the Knockout library we can create some Java Script file which can look like below:
Next, it is necessary to create the HTML and add data binding between the view and the view model (JavaScript).
Sample HTML file to display data with using Web API can be like:
Finally the launched application should look like:
Conclusion
In this article I presented how to create a sample Web API service which can be used on various clients.
I used the ASP.NET Web API 2 with Entity Framework 6 to create a simple web application that communicate with back-end database.
Author: Sebastian Pisulski
back